What is if __name__ == "__main__" in Python

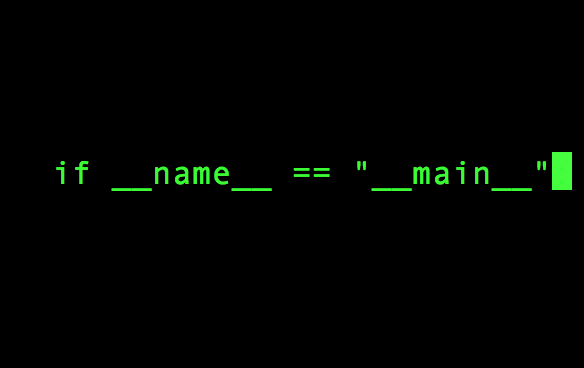
Python is one of the growing high level languages out there. That claim is not without proof. In the recent list of language popularity published by GitHub, Python was in the top list. In fact it is the second most popular language (the first place is currently held by JavaScript). The syntax of python is quite straight forward and easy to understand, without the confusing curly braces all over the place.
Another reason for Python's popularity is its use as a server side scripting language. System administrators can leverage Python to get their boring routine work automated. With the availability of ready made modules for almost everything, its quite easy to implement something in Python these days.
In this article we will discuss one of the popular statements that new python users encounter in the programs. Its below.
if __name__ == "__main__":
We will be discussing the use case and reason for the above statement in programs.
One of the main factor that made programming easy and attractive is "Modules". Module in Python is nothing but a program that can be included(imported) in other programs, so that the functions and operations defined in the module can be reused in the program that you are writing.
To understand it further lets write a small program and import it as a module in another program. Below is a small program with a dummy function that prints something. We will import this program as a module in another program.
#cat myfirstmodule.py def printsomething(): print "Hello!. Welcome!"
Lets write another dummy program that will import the above program. Remember that the above dummy program is saved in a file named "myfirstmodule.py", so when we import it to our program, we will simply say "import myfristmodule". See below.
#cat myfirstprogram.py import myfirstmodule myfirstmodule.printsomething()
Now if you execute myfirstprogram.py, it should execute the function "printsomething" defined in "myfirstmodule". You can access all functions defined in myfirstmodule using the format "myfirstmodule.functioname" inside any program where you have imported it.
$python myfirstprogram.py Hello!. Welcome!
Let's now include one more line inside our first module named "myfirstmodule.py". See below.
#cat myfirstmodule.py def printsomething(): print "Hello!. Welcome!" printsomething()
We simply called the printsomething function to see if it working as expected inside myfirstmodule. Now let's again execute myfirstprogram.py. See below.
$python myfirstprogram.py Hello!. Welcome! Hello!. Welcome!
We now see an undesired output of two print statements. One is coming from the "printsomething()" line we added inside "myfirstmodule.py" and the second is coming from the line "myfirstmodule.printsomething()" inside myfirstprogram.py.
This is because when python encounters the import statement ("import myfirstmodule" in our case), it executes it, so that the functions and other attributes are then available to the current program where you are importing it. In our example, when python interpreter saw "import myfirstmodule", it executed it, which also contained the new line that we added (printsomething())
The printsomething() line we added inside was just to test our function. It should not be executed when "myfirstmodule" is imported as a module. It should only be executed when myfirstmodule.py is executed directly (ie: python myfirstmodule.py)
Lets now add one more line to our module. See below.
#cat myfirstmodule.py def printsomething(): print "Hello!. Welcome!" if __name__ == "__main__": printsomething()
Lets again execute myfirstprogram.py. It should not generate the undesired output of two print statement we saw earlier. Remember that we did not touch myfirstprogram.py at all. We only modified myfirstmodule.py with a magic if statement if __name__ == "__main__"
$python myfirstprogram.py Hello!. Welcome!
The line if __name__ == "__main__": tells the program that the code inside this if statement should only be executed if the program is executed as a standalone program. It will not be executed if the program is imported as a module.
But how does that work. Lets dig a bit more deeper.
Let's see what is __name__ in a program. How can we verify what is __name__?. Lets start python interpreter in the same location where we have myfirstmodule.py and myfirstprogram.py. Python interpreter can be started by simply executing the command python. See below.
$ python Python 2.7.10 (default, Jul 14 2015, 19:46:27) [GCC 4.2.1 Compatible Apple LLVM 6.0 (clang-600.0.39)] on darwin Type "help", "copyright", "credits" or "license" for more information. >>>
Now lets see what is the value of __name__ in Python.
>>> >>> print __name__ __main__ >>>
So basically...__name__ is by default set to the value of __main__ in python.. But lets see importing a module and then see the value of __name__ inside that module. See below(remember, we are inside python interactive interpreter in the same location where we have myfirstprogram.py and myfirstmodule.py).
>>> >>> import myfirstmodule >>> print myfirstmodule.__name__ myfirstmodule >>> >>>
So the __name__ variable now has the value of "myfirstmodule". Any program in python that is imported as a module will have __name__ variable set to the name of the module. In all other cases it will be the default value of __main__
When myfirstmodule is executed from another program as a module, then the value of __name__ becomes "myfirstmodule" (ie: the name of the module). When myfirstmodule is executed as a standalone program, __name__ will have the default value of __main__.
Here is the bottom line: Whenever you need a program, that needs to be executed as a script/standalone program, and you would also like to import it inside other programs, then you use if __name__ == "__main__":
Things that needs to be executed in standalone mode will go inside the if statement block if __name__ == "__main__":
Comments
Thank you
Best tutorial i have come across from ever since i started programming!!thank you sir, do more of this
Superb!....clear and detailed
Superb!....clear and detailed explanation...
Add new comment